Hibernate and PostgreSQL configuration with Maven
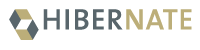
In this tutorial I am going to show you how to configure connection between Hibernate and PostgreSQL in Java application using Maven.
I am using:
JDK 1.7.0_72
Apache Maven 3.2.1
PostgreSQL 9.3.5
Hibernate 4.2.15.Final
1. Generate maven project from archetype
mvn archetype:generate -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false -DgroupId=com.jvmhub.tutorial -DartifactId=01-hibernate-conf
2. Add fallowing dependencies to you pom.xml file
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.jvmhub.tutorial</groupId> <artifactId>01-hibernate-conf</artifactId> <packaging>jar</packaging> <version>1.0-SNAPSHOT</version> <name>01-hibernate-conf</name> <properties> <hibernate.version>4.2.15.Final</hibernate.version> </properties> <dependencies> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-entitymanager</artifactId> <version>${hibernate.version}</version> </dependency> <!-- The tutorials use the PostgreSQL 9.3.5 database --> <dependency> <groupId>org.postgresql</groupId> <artifactId>postgresql</artifactId> <version>9.3-1102-jdbc41</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> </project>
3. Create directory resources in src/main and add hibernate.cfg.xml file.
<?xml version='1.0' encoding='UTF-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Connection settings --> <property name="hibernate.connection.driver_class">org.postgresql.Driver</property> <property name="hibernate.connection.url">jdbc:postgresql://localhost/jvmhubtutorial</property> <property name="hibernate.connection.username">user</property> <property name="hibernate.connection.password">password</property> <!-- SQL dialect --> <property name="hibernate.dialect">org.hibernate.dialect.PostgreSQLDialect</property> <!-- Print executed SQL to stdout --> <property name="show_sql">true</property> <!-- Drop and re-create all database on startup --> <property name="hibernate.hbm2ddl.auto">create-drop</property> <!-- Annotated entity classes --> <mapping class="com.jvmhub.tutorial.entity.AppUser"/> </session-factory> </hibernate-configuration>
This file contain configuration of connection with PostgreSQL database.
3. Create a simple entity classAppUser for persistence mapping
package com.jvmhub.tutorial.entity; import java.io.Serializable; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class AppUser implements Serializable { public AppUser() { }; public AppUser(String login) { this.login = login; }; @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String login; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getLogin() { return login; } public void setLogin(String login) { this.login = login; } }
Warning! User is a keyword in PostgreSQL so you may have problems with creating that table in you database.
4. Check working of this connection by simple test and then execute mvn test command in the project’s directory.
package com.jvmhub.tutorial; import junit.framework.TestCase; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; import com.jvmhub.tutorial.entity.AppUser; /** * Unit test for simple App. */ public class AppTest extends TestCase { public void testApp() { SessionFactory sessionFactory = new Configuration().configure() .buildSessionFactory(); Session session = sessionFactory.openSession(); session.beginTransaction(); AppUser user = new AppUser("firstuser"); session.save(user); session.getTransaction().commit(); session.close(); } }
5. After that all you should have directory structure like below:
If everything is OK, you should have your first entry in the database persist by Hibernate!
Complete source code: https://github.com/jvmhub/Hibernate-and-PostgreSLQ-configuration-with-Maven
Pingback: Hibernate and PostgreSQL configuration using persistence.xml and EntityManager | Java Web Development Blog